Personal Views / Basic Principles
[IMO] 编程是一件偏主观的事 is very subjective
- 比起数学公式它更像是论文 或者 有些人可以写成散文
- Engineering rather than Science
- 如果让计算机自己学习,那我们的工作就更像是艺术家 Deep Learning is often considered more of an art than a science because it requires a lot of experimentation and trial-and-error to achieve optimal results.
简单暴力 KISS (Keep it simple, stupid)
[IMO]Clarity First,Optimization second. 能让人看懂是最优先, 性能再好都是其次。
[IMO] 尽量别用显式的去用Java的多线程,很难不出错。很难debug
- 为啥要用, 异步 & 性能(利用多核)- 用框架去解决。netty akka erlang exilir
- 如果你一定要用, 1.把你写的并行代码和其他代码隔离开,单独弄个module。 2 使用ExecutorService。
ExecutorService abstracts away many of the complexities associated with the lower-level abstractions like raw Thread
任何人都能写出workable的代码,好程序员写出来的是人类能懂的 “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” — Martin Fowler**
著名的雷神之锤代码 Q_rsqrt https://github.com/id-Software/Quake-III-Arena/blob/master/code/game/q_math.c
one of our recent candidate’s code
1 | public static int[] transform(int [] array){ |
we can do better than this?
1 |
|
快速试错 Rapid Iterative Testing and Evaluation (RITE)
- 找到问题的根源,在根源上改。 think before you code, don’t make adhoc change or tweak some if condition without much thinking
- 重复不利于试错 不收敛,程序员最懒惰,工作为了不工作 DRY (Don’t Repeat Yourself) principle
- 一切都是代码,ut和ft也是代码需要review.”Talk is cheap. Show me the code.” – Linus Torvalds
没有绝对的正确,核心是我们怎么妥协 “There are no solutions, only tradeoffs - Thomas Sowell”
- 做一个有态度的 reviewer - Having a clear direction helps us converge quickly.
- code review 包含了主观的偏好 subjective [IMO] objective[FACT]
- senior 一定是对的吗 I disagree with your disagreement
- Let’s appeal to rules that all team member respect (We Make Up the Rules as We Go Along)
- 做一个有态度的 reviewer - Having a clear direction helps us converge quickly.
Replace Conditional with Polymorphis
- 什么是条件表达
- if elseif else, switch case
- 为什么条件表达多了不好
无法一眼看清 nested condition 更差
不好修改, 修改太分散(shotgun surgery 散弹式修改)有时需要在好几个else if里面加同样的代码
方法容易写太长, 这样方法也难复用了
how to make it better
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15class Employee {
int payAmount(RoleType type){
switch(type){
case ENGINEER:
return getMonthlySalary();
case SALESMAN:
return getMonthlySalary() + getMonthlyCommission();
case MANAGER:
return getMonthlySalary() + getBonus();
default:
throw new RuntimeException("Invalid employee role type");
}
}
}
it works but we can do better:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20abstract class Employee{
abstract int payAmount();
}
class Engineer extends Employee{
int payAmount(){
return getMonthlySalary();
}
}
class Salesman extends Employee{
int payAmount(){
return getMonthlySalary() + getMonthlyCommission();
}
}
class Manager extends Employee{
int payAmount(){
return getMonthlySalary() + getBonus();
}
}
Improve Conditional Expression
- prefer positive checks
- IsEmpty() NOT IsNotAllEmpty()
- hasContent() NOT HasNotAnyContent()
- use gaurd and fail fast
- [NOT] garbage in , garbage out.
- throw error and exception, stop return.
- 每个方法只做一件事,这么多if是不是因为想做的太多
- 也有可能是level 太多, 把低level的abstract出去,形成一个方法。 比如和persist相关的。和mq相关的
- 3层最多了 别再多了
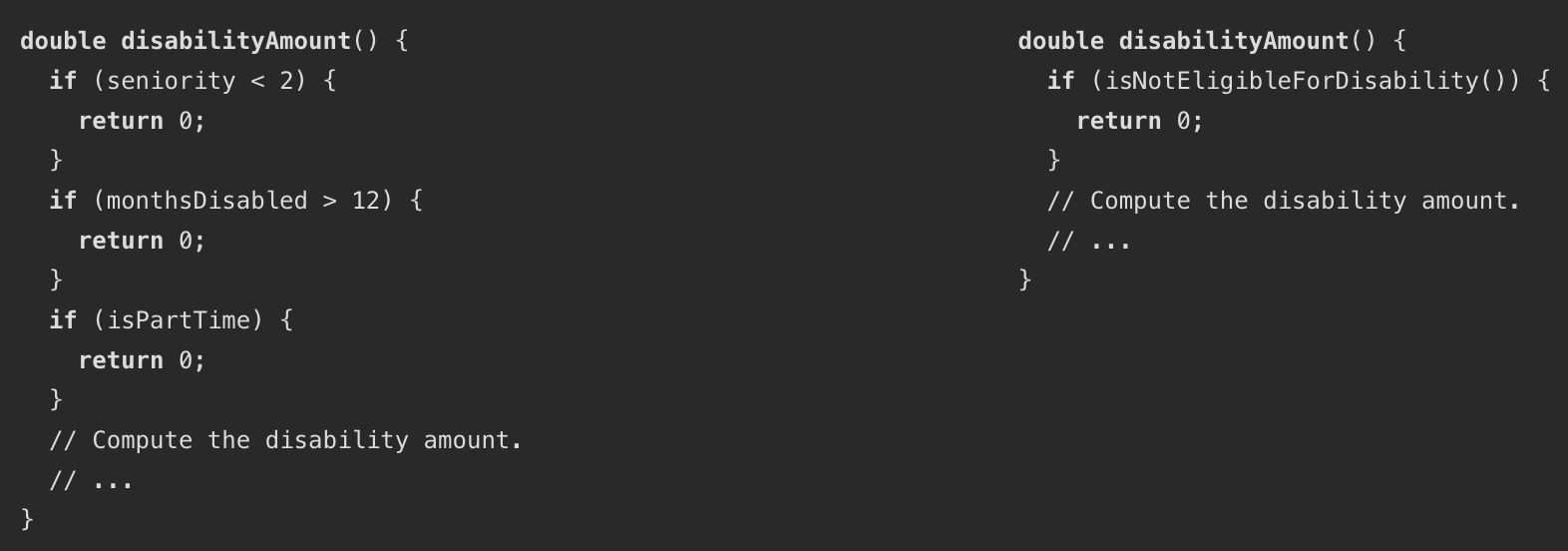
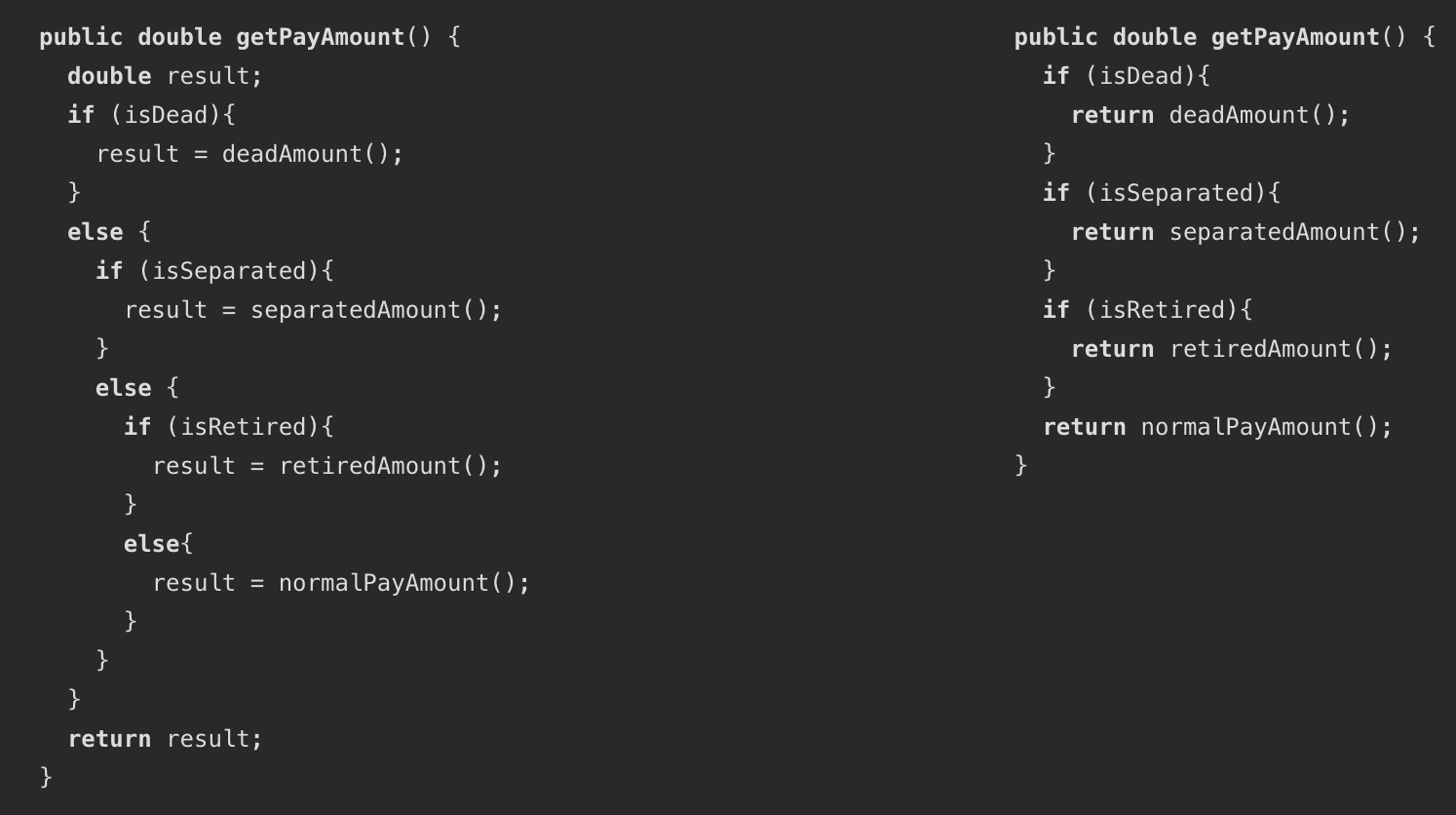
Be proactive
- 主动去学,既然像写文章。那肯定是多看好的 少看bad code。看多了你也开始这么写了。
- 那去看什么?
大牛写的不适合你 他们的代码也很底层 你模仿不来
github上高星的项目都是大公司开源的可以通用的工具或平台 kafka spark flink。你写业务代码和这些优秀的强关注performance的项目 目标就不太一致
那看点儿啥?你的代码是搭载什么上边的 你和平台的风格靠拢。 你在别人的项目相关下工作, 和别人写的风格靠拢。别突出自己的不同。
https://github.com/spring-projects/spring-petclinic spring 自己提供的一个他们觉得要这么写比较的好的 事例应用。宠物诊所
Spring Music(https://github.com/cloudfoundry-samples/spring-music) :一个使用Spring Boot构建的音乐存储和检索示例应用程序。
Spring RESTbucks(https://github.com/restbucks/restbucks-java-spring) :一个使用Spring Boot构建的咖啡订购和配送系统示例。
Spring Trader(https://github.com/spring-projects/spring-trade) :一个使用Spring Boot构建的股票交易示例程序,展示了金融交易相关的业务逻辑。
Spring PetClinic Microservices(https://github.com/spring-petclinic/spring-petclinic-microservices): Spring PetClinic的微服务版本,它将业务功能划分为多个微服务。
Extensive Reading
https://github.com/google/styleguide/tree/gh-pages
https://github.com/alibaba/Alibaba-Java-Coding-Guidelines#naming-conventions